
Get access to Intro to Full Stack Javascript
- Practical, go-at-your-own pace learning, with help from our industry experts and experienced teachers
- Projects and code that you can alter and include in your own sites and portfolio
- Resources to get you started and going post-course
Lesson 12
Walkthrough: Adding a form and animating our AddNote component
A Walkthrough of our previous Challenge so that we’re all on the same page.
Our final AddNote component:
import { useRef, useState, useEffect } from "react";
import { Add, Title, TitleInput, BodyInput, Form, Button } from "./styles";
import { Formik, FormikHelpers } from "formik";
import { useSpring } from "react-spring";
interface IProps {
openAddNote: boolean;
setOpenAddNote: React.Dispatch>;
folder: string;
setNoteIsAdded: React.Dispatch>
}
const AddNote: React.FC = ({ openAddNote, setOpenAddNote, folder, setNoteIsAdded }) => {
const newNoteRef = useRef(null);
const [noteHeight, setNoteHeight] = useState(50);
// useEffect to set height
useEffect(() => {
setNoteHeight(
newNoteRef.current
? openAddNote
? newNoteRef.current.scrollHeight
: 40
: 40
);
}, [openAddNote]);
// Types
interface FormValues {
title: string;
body: string;
}
// Submit
const handleSubmit = (
values: FormValues,
{ setSubmitting, resetForm }: FormikHelpers
) => {
setTimeout(() => {
setSubmitting(false);
const currentFolder = localStorage.getItem(folder);
const notes = currentFolder ? JSON.parse(currentFolder) : [];
const newNoteAdded = [values.title, values.body];
notes.push(newNoteAdded);
const updatedNotes = JSON.stringify(notes);
localStorage.setItem(folder, updatedNotes);
handleToggle();
setNoteIsAdded(true)
resetForm();
}, 400);
};
// Animation props
const addNoteProps = useSpring({
maxHeight: noteHeight,
config: openAddNote
? { duration: 300, tension: 10, friction: 10 }
: { duration: 400, tension: 10, friction: 10 },
});
// Toggle
const handleToggle = () => {
setOpenAddNote(!openAddNote);
};
return (
<>
+ Add note
{openAddNote && (
{
const errors: Partial = {};
if (!values.title) {
errors.title = "Title is required";
}
return errors;
}}
onSubmit={handleSubmit}
>
{({ values, handleChange, handleSubmit }) => (
)}
);
};
export default AddNote;
Private notes
A place for you to post notes about anything on this page. Only you can view your notes.
SuperHi FM
Want some ambient music in the background? Play our radio station!
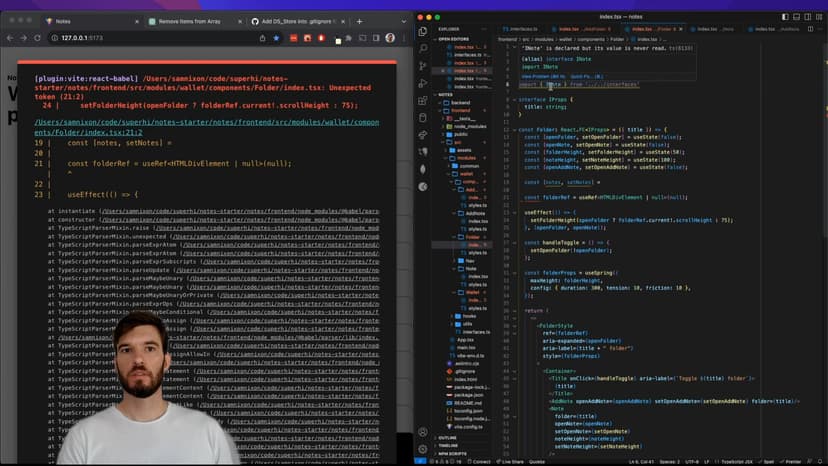
Next: Lesson 13
Iterating through our wallet
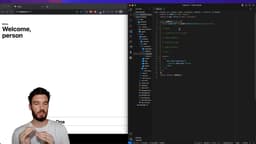
Back: Lesson 11
Challenge: Adding a form and animating our AddNote component
Feeling stuck?
Don’t worry, we are here to help you with:
- Speedy help from one of our team members
- Detailed, relevant solutions
- Direct access to peer support through Discord!
Remember, there’s no such thing as a silly question, so don’t hesitate to reach out, we love hearing from you!